Async Await: How the heck do I use this thing?
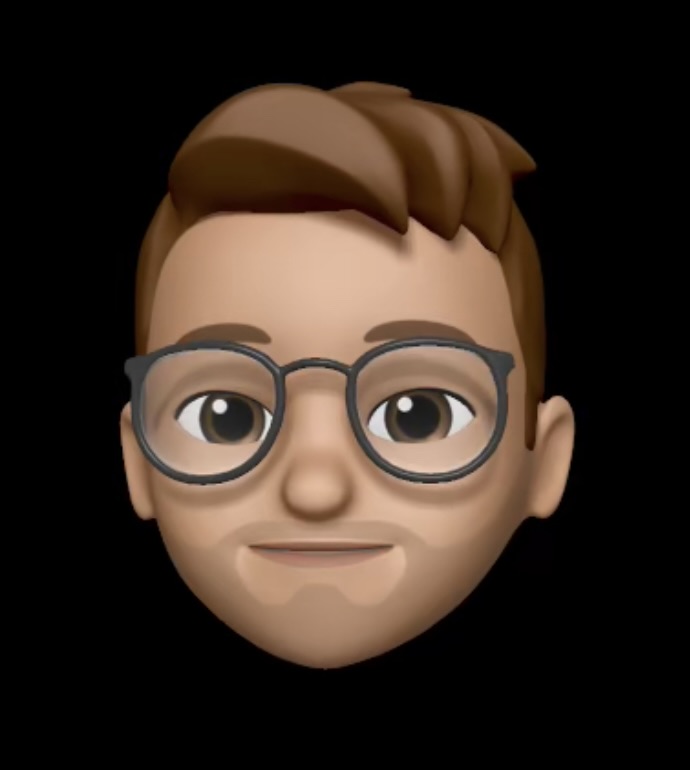
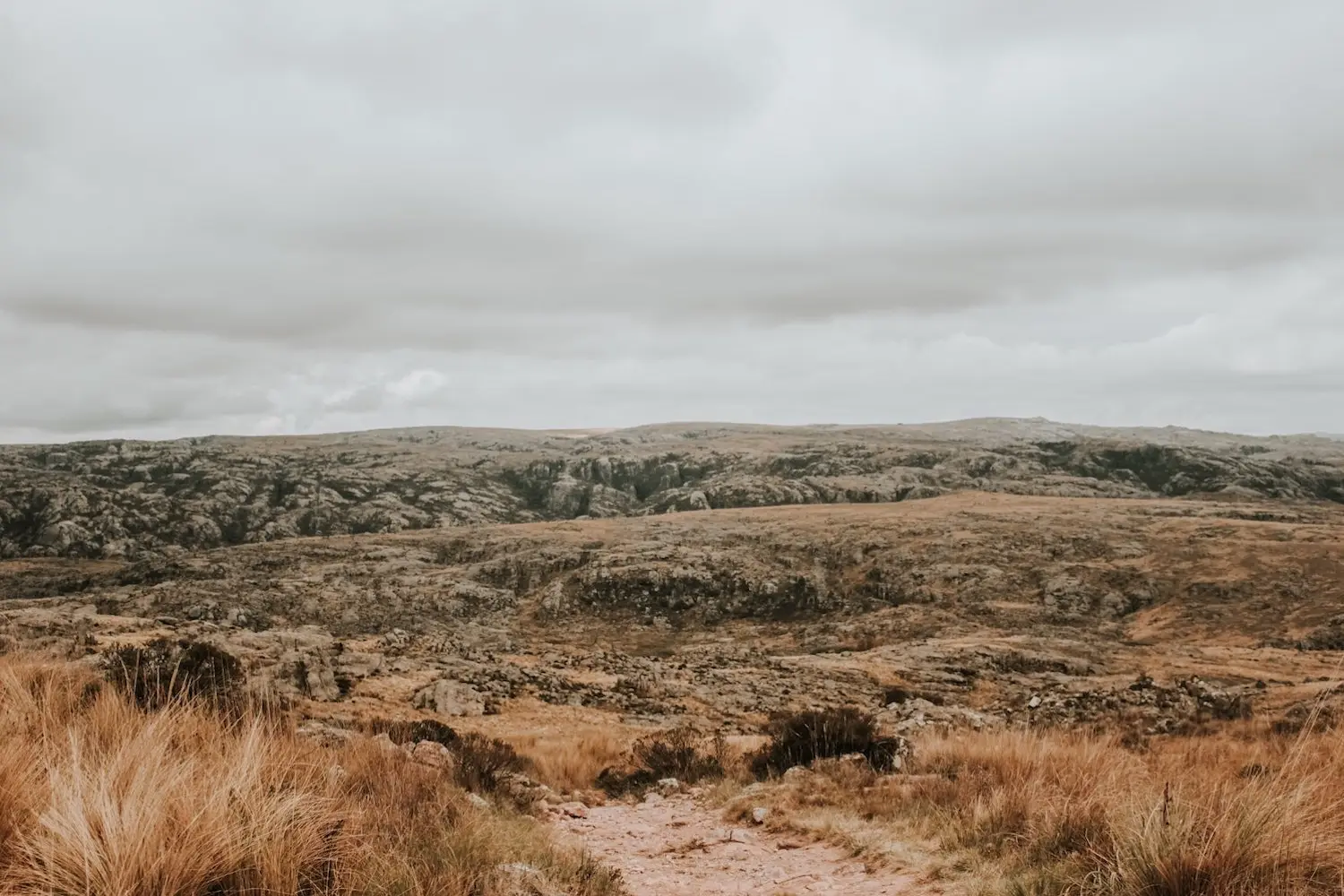
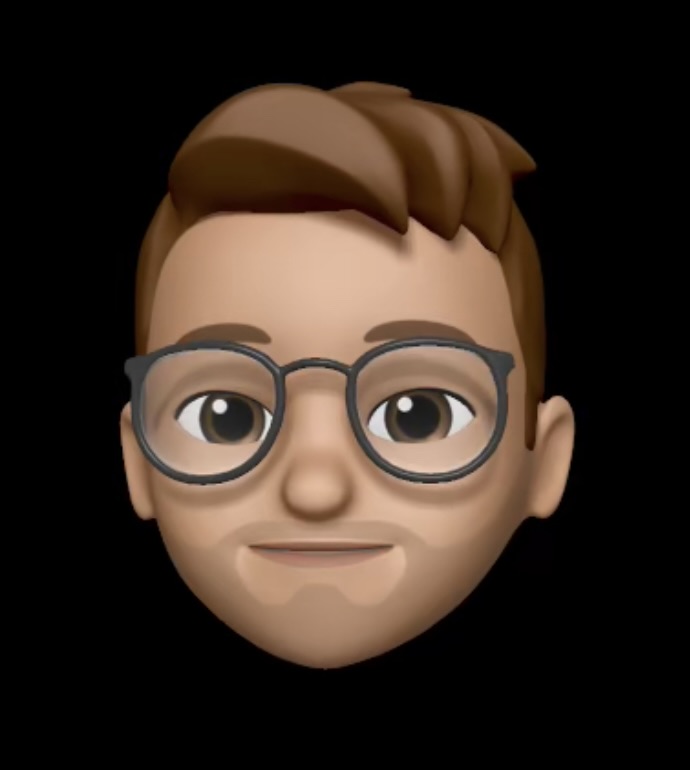
If you're like me, you've heard of Async Await, and after reading numerous articles and trying to piece it all together, you were left with more questions than answers. So today I hope to give you a better understanding of the concepts!
So to get started, we should start by saying that async and await are extensions of promises. I wrote an article recently over promises, and it can be found here. The MDN documentation gives a brief description of an async function as the following:
An async function is a function declared with the async keyword, and the await keyword is permitted within it. The async and await keywords enable asynchronous, promise-based behavior to be written in a cleaner style, avoiding the need to explicitly configure promise chains.
Lets go over this some more, and how to use it!
Why is Async Await helpful, and how do we use it?
Async Await gives us better control of what will happen in our code. Say you had to perform some functionality, and wanted to write a series of functions that relied on one another for completion, you could have the functions use async await to handle the sequential operation of things. This in turn will make sure the code works as expected and things don't break.
To show how this works, we will start with the most basic async function and build on top of it. We can take a function, and append the async keyword onto it. The word "async" before a function tells it to do one simple thing, which is to always returns a promise. Remember, an async function ALWAYS returns a promise.
async function returnValue() {
return 1;
}
Another way to write the code above would be
async function returnValue() {
return Promise.resolve(1);
}
So, async makes sure our function will return a promise, and will wrap non promises in it. Pretty cool, huh? On top of that, we have another keyword, which only works inside an async function, and that keyword is await.
Lets go over an example, and extend upon the original async function above
async function returnValue() {
let ourReturnedValue = new Promise((resolve, reject) => {
setTimeout(() => resolve(1), 1000)
});
let ourResult = await ourReturnedValue;
console.log(ourResult); // output: 1
}
While we typically want to avoid setTimeouts, for this example it would be fine.
I bet you're wondering what would happen if we tried throwing an await inside of a normal function. Lets go over that
function returnValue() {
let ourPromise = Promise.resolve(1);
let ourResult = await promise;
}
With this, what would you expect to happen? If you guessed that a syntax error is thrown, you're correct. With this, we get the error
Uncaught SyntaxError: await is only valid in async functions and the top level bodies of modules
But why is this happening? Well, as previously discussed, the await keyword is only available inside of an async function. Since we don't have access to await inside of our normal function, we get an error.
How do we handle errors?
Since errors should be handled appropriately, there are a few different ways we could write in our error handling
We could could do a promise.reject()
async function throwAnError() {
await Promise.reject(new Error("This is an error!"));
}
We could explicitly call a throw
async function throwAnError() {
throw new Error("This is an error!");
}
We could use a try catch
async function returnValue() {
try {
let ourReturnedValue = new Promise((resolve, reject) => {
setTimeout(() => resolve(1), 1000)
});
let ourResult = await ourReturnedValue;
} catch(error) {
console.log(error);
}
}
We use the call of the promise generated by the the async function, as it becomes rejected
async function returnValue() {
let ourReturnedValue = new Promise((resolve, reject) => {
setTimeout(() => resolve(1), 1000)
});
let ourResult = await ourReturnedValue;
console.log(ourResult);
}
returnValue().catch(alert);
When using async await , we should rarely need to use then , as the await will handle all of the waiting. Whether or not to use a try/catch versus a catch is up to you, but I tend to lean more toward the former, as I find it easier to read and follow. With that being said, it's really up to you and your personal preferences.
Thank you for reading, and have a good one!